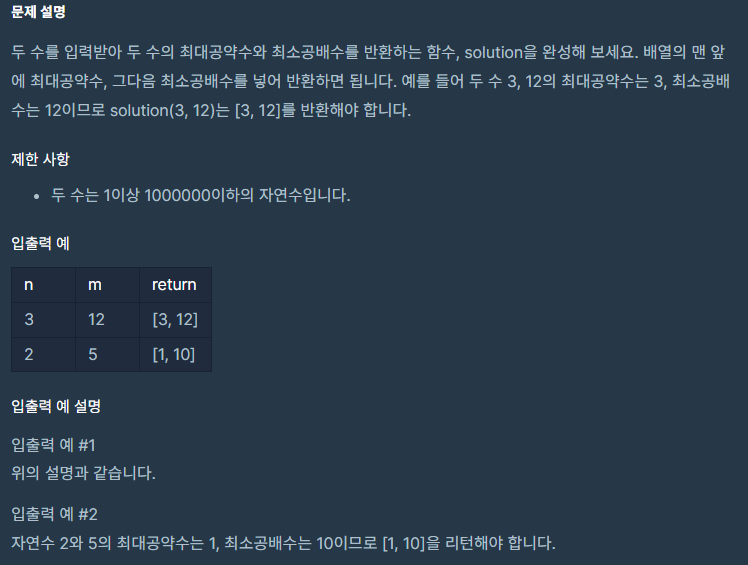
프로그래머스 C# 최대공약수와 최소공배수
·
알고리즘
문제 해결방안public class Solution { public int[] solution(int n, int m) { int[] answer = new int[] {}; int min = GCD(n,m); int max = LCM(n,m,min); answer = new int[]{min,max}; return answer; } int GCD(int a_, int b_) { if (b_ == 0) return a_; else return GCD(b_, a_ % b_); } int LCM(int a_, i..